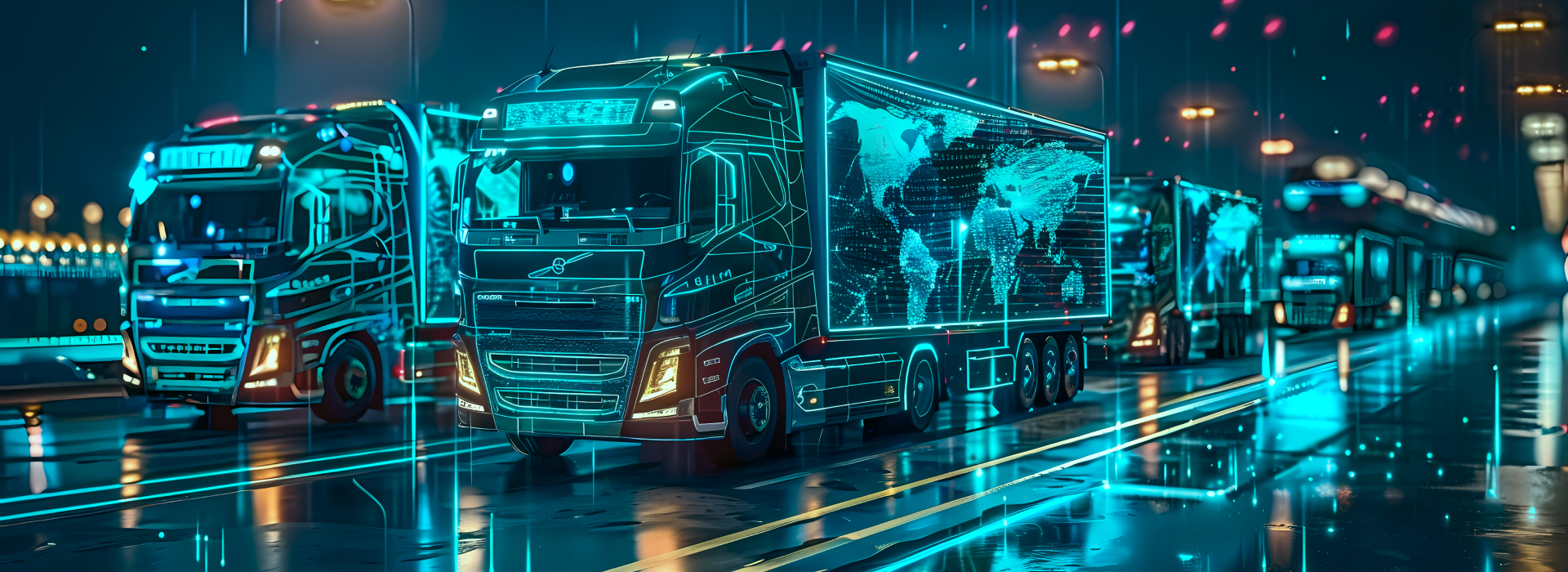
MDM for Logistics: Empower Your Business with Efficient Mobile Device Management Software
We reveal business benefits of using MDM for logistics. Discover how centralized device management ensures data security and simplifies the IT routine...
We reveal business benefits of using MDM for logistics. Discover how centralized device management ensures data security and simplifies the IT routine...
Buy Now Pay Later (BNPL) solution development: market overview, must-have features, technology stack, and implementation challenges
Learn to develop custom file system minifilter drivers and prevent unauthorized access with our practical step-by-step guide.
Discover how to create a custom AI chatbot for your business and why it requires significant time, expertise, and investment to develop a high-quality...
Discover how big data helps FinTech projects with tasks ranging from detecting fraud to personalizing services. Explore real-world implementation chal...
Learn how to create a DeFi app along with details on key functionalities, the technology stack, development challenges, and DeFi trends.
Discover how to integrate fintech APIs efficiently. Learn key considerations for security, compliance, and performance to optimize your fintech soluti...
We walk you through how to create a digital wallet step by step and discuss wallet types, must-have features, and business benefits.
Explore the key risks to embedded system security, the attacks embedded systems suffer from most, and Apriorit’s expert tips to protect embedded sys...
Explore Apriorit’s overview of warehouse drone management system development, from use cases and features to app architecture and tech stack.
Tell us about
your project
...And our team will:
Do not have any specific task for us in mind but our skills seem interesting? Get a quick Apriorit intro to better understand our team capabilities.